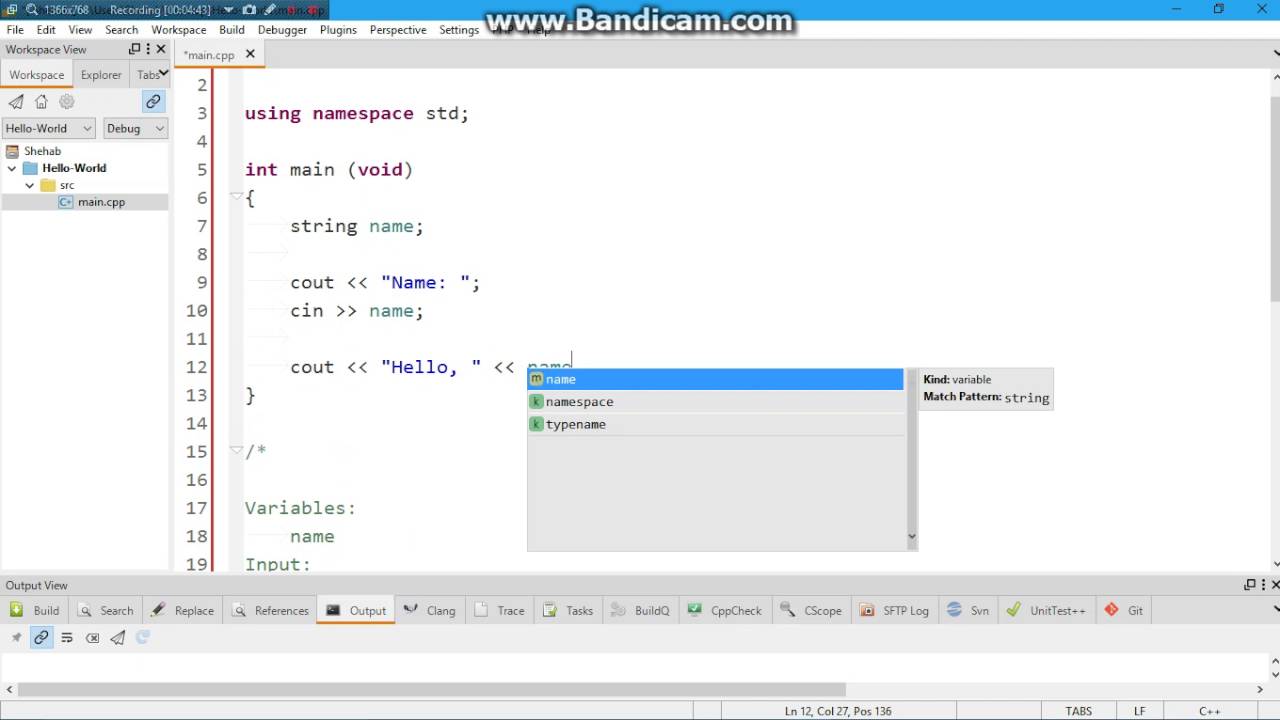
Taking Input from the User in C++ YouTube
3 I am trying to read an integer from terminal. Here's my code: int readNumber () { int x; std::cin >> x; while (std::cin.fail ()) { std::cin.clear (); std::cin.ignore (); std::cout << "Bad entry. Enter a NUMBER: "; std::cin >> x; } return x; } Whenever I run this code I get:
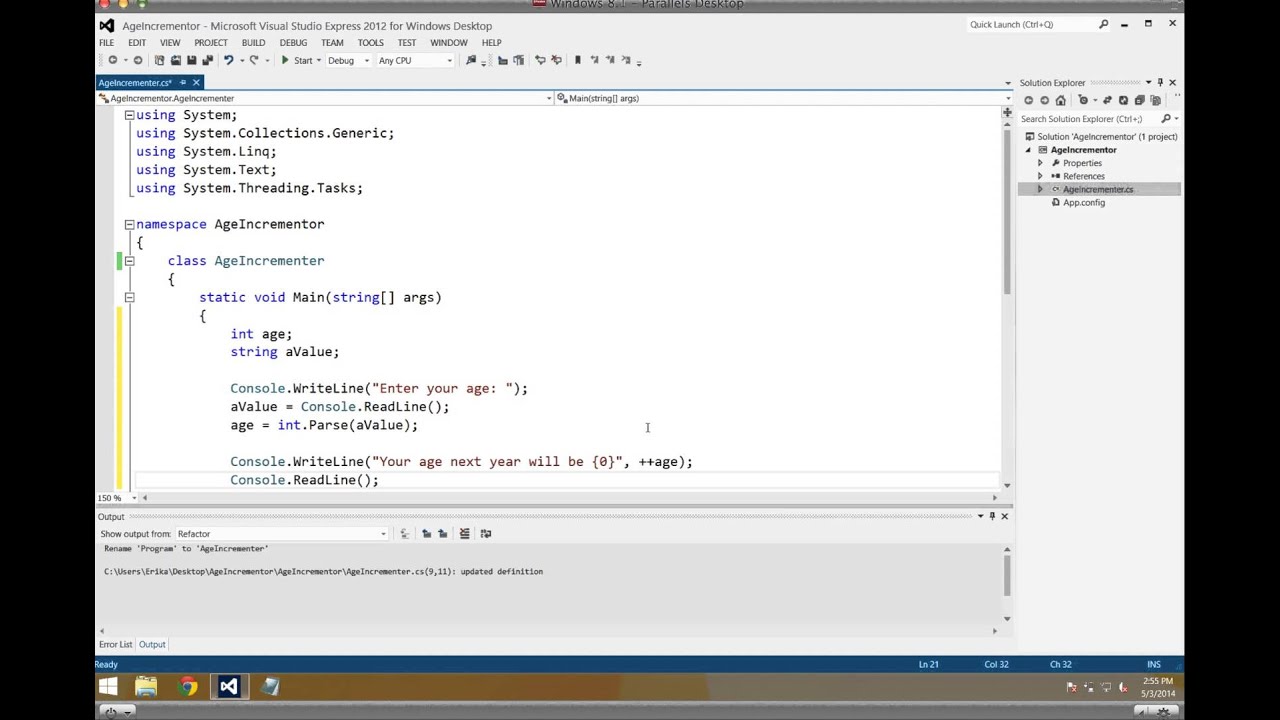
User Input and Converting a String to an Integer in C YouTube
How to take input and output of basic types in C? The basic type in C includes types like int, float, char, etc. Inorder to input or output the specific type, the X in the above syntax is changed with the specific format specifier of that type. The Syntax for input and output for these are: Integer:
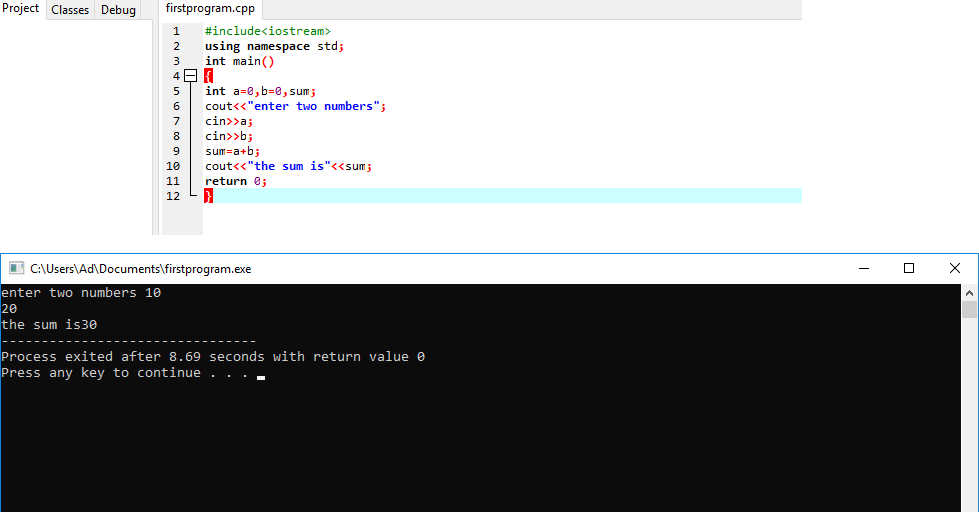
input output in c++ Sharp Tutorial
The given task is to take an integer as input from the user and print that integer in C++ language. In this article, we will learn how to read and print an integer value. In the below program, the syntax and procedures to take the integer as input from the user is shown in C++ language. Standard Input Stream
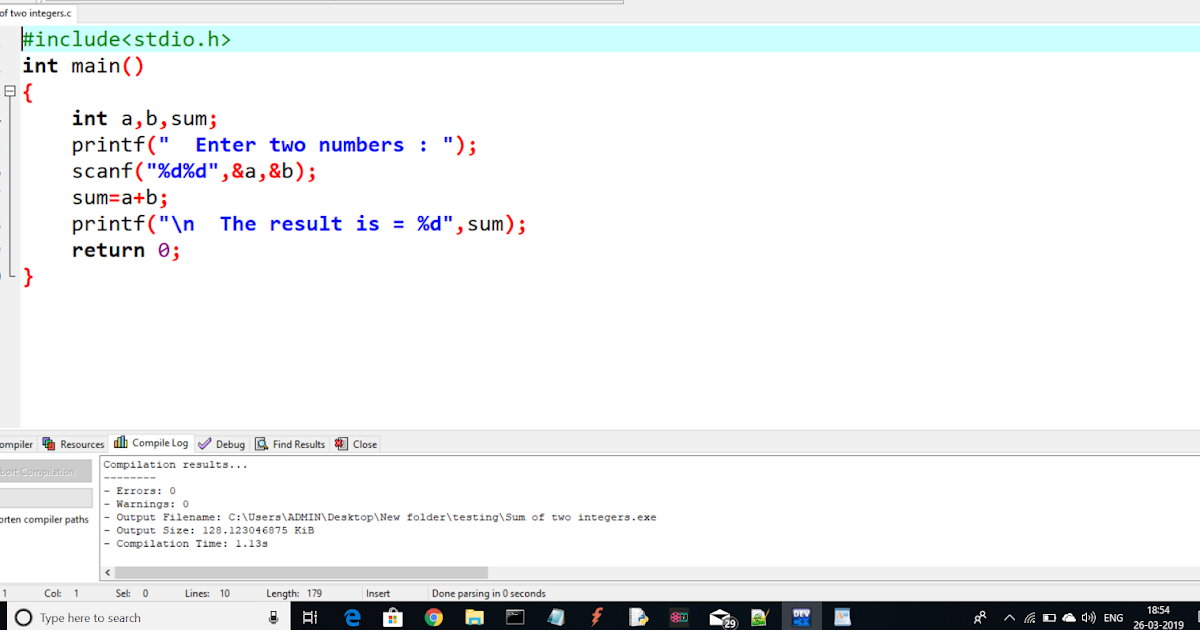
Basic Integer Addition in C language
To get user input, you can use the scanf () function: Example Output a number entered by the user: // Create an integer variable that will store the number we get from the user int myNum; // Ask the user to type a number printf ("Type a number: \n"); // Get and save the number the user types scanf("%d", &myNum); // Output the number the user typed
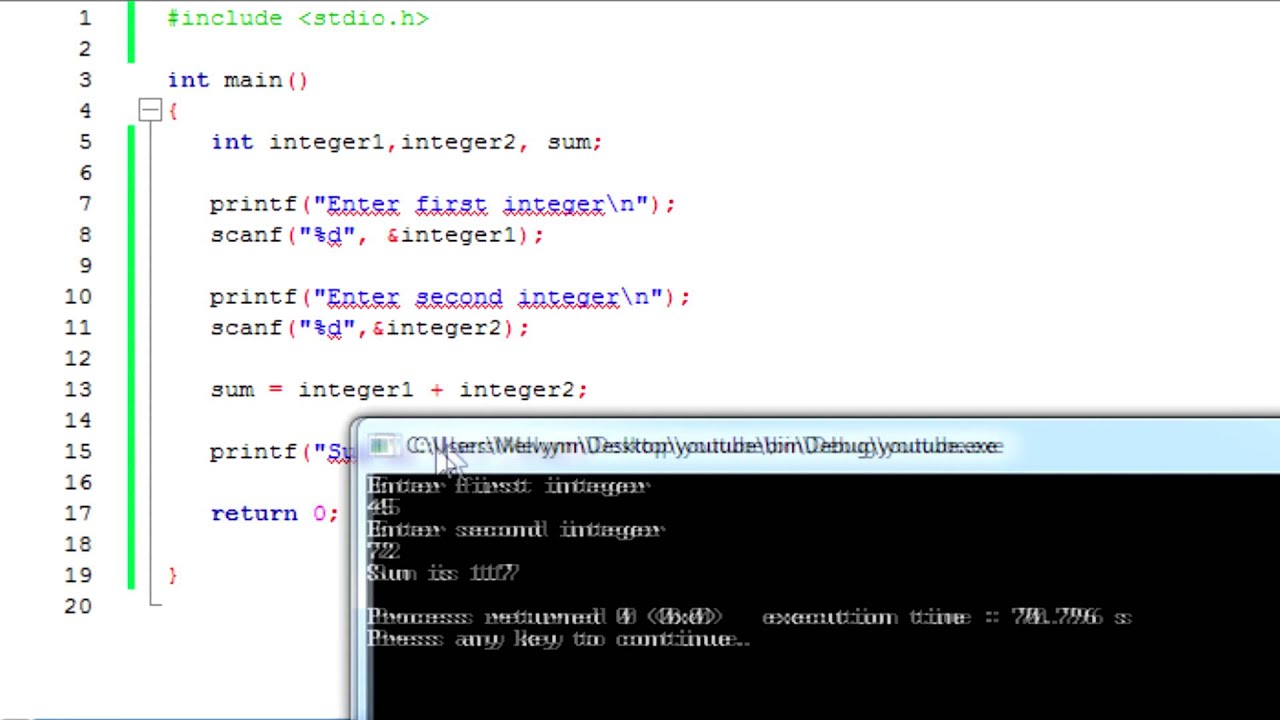
2 C Program for adding integers / arithmetic operators in C YouTube
The printf () is a library function to send formatted output to the screen. The function prints the string inside quotations. To use printf () in our program, we need to include stdio.h header file using the #include
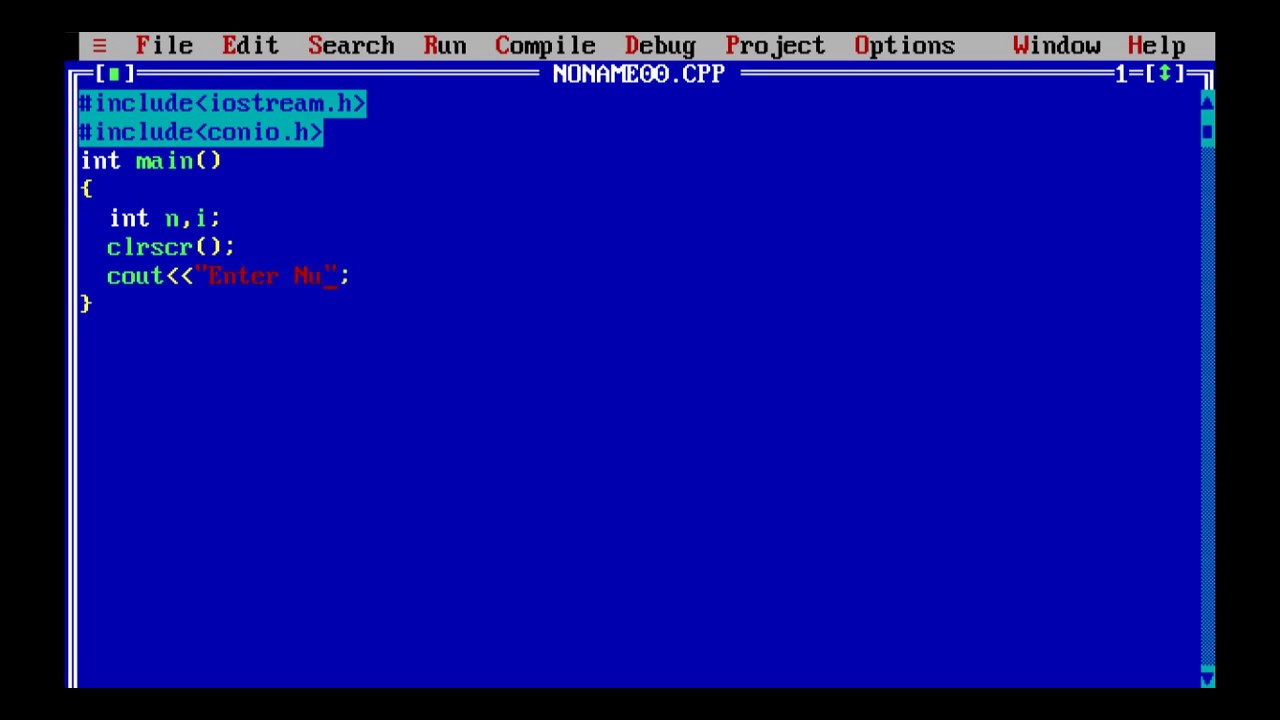
10. Write a C++ program to input an integer number and display on screen "Well Done" as many
C Program to read and print an Integer, Character and Float using scanf and printf function. This program takes an integer, character and floating point number as input from user using scanf function and stores them in 'inputInteger', 'inputCharacter' and 'inputFloat' variables respectively. Then it uses printf function with %d, %c.
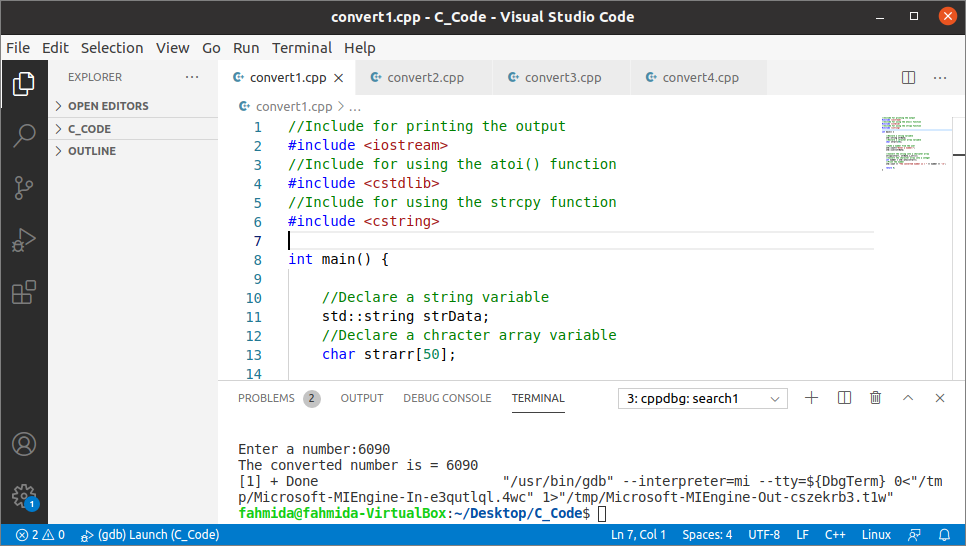
How to convert a string into an int in C++
// Type your username and press enter Console.WriteLine("Enter username:"); // Create a string variable and get user input from the keyboard and store it in the variable string userName = Console.ReadLine(); // Print the value of the variable (userName), which will display the input value Console.WriteLine("Username is: " + userName); Run example »
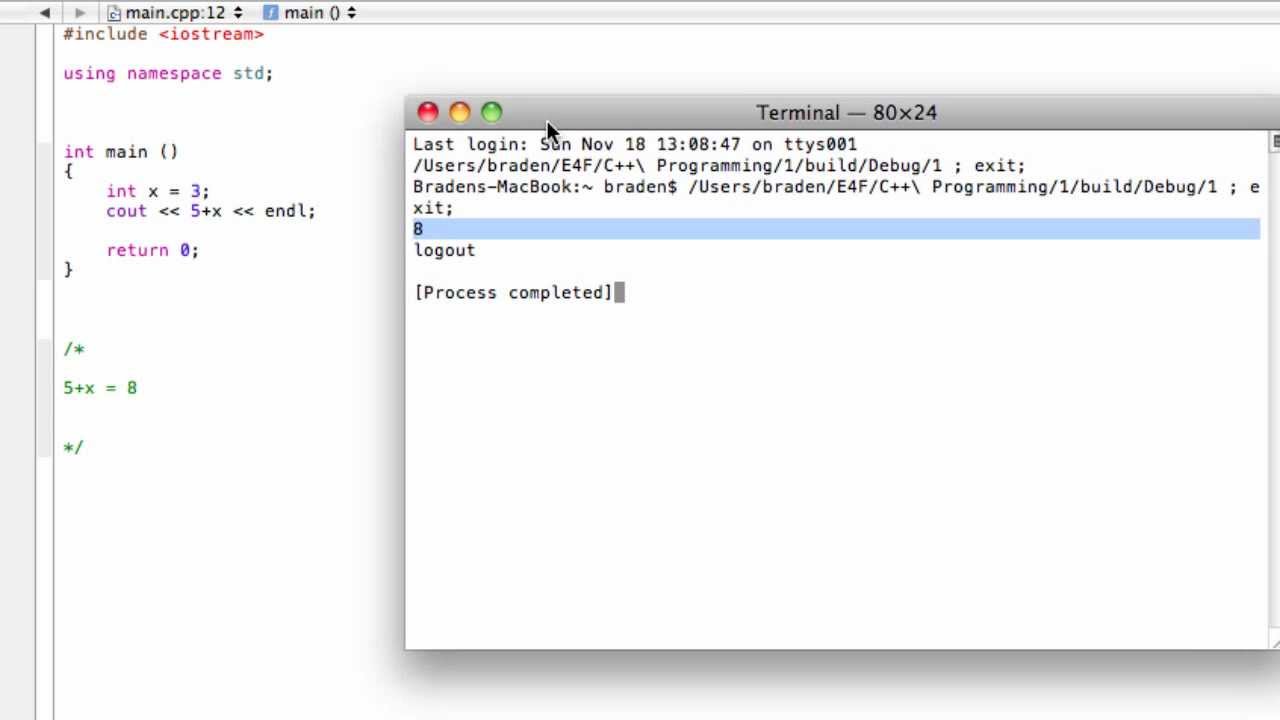
Using the int variable (C++ programming tutorial) YouTube
The double quoting is what makes the difference; when the text is enclosed between them, the text is printed literally; when they are not, the text is interpreted as the identifier of a variable, and its value is printed instead. For example, these two sentences have very different results: 1 2
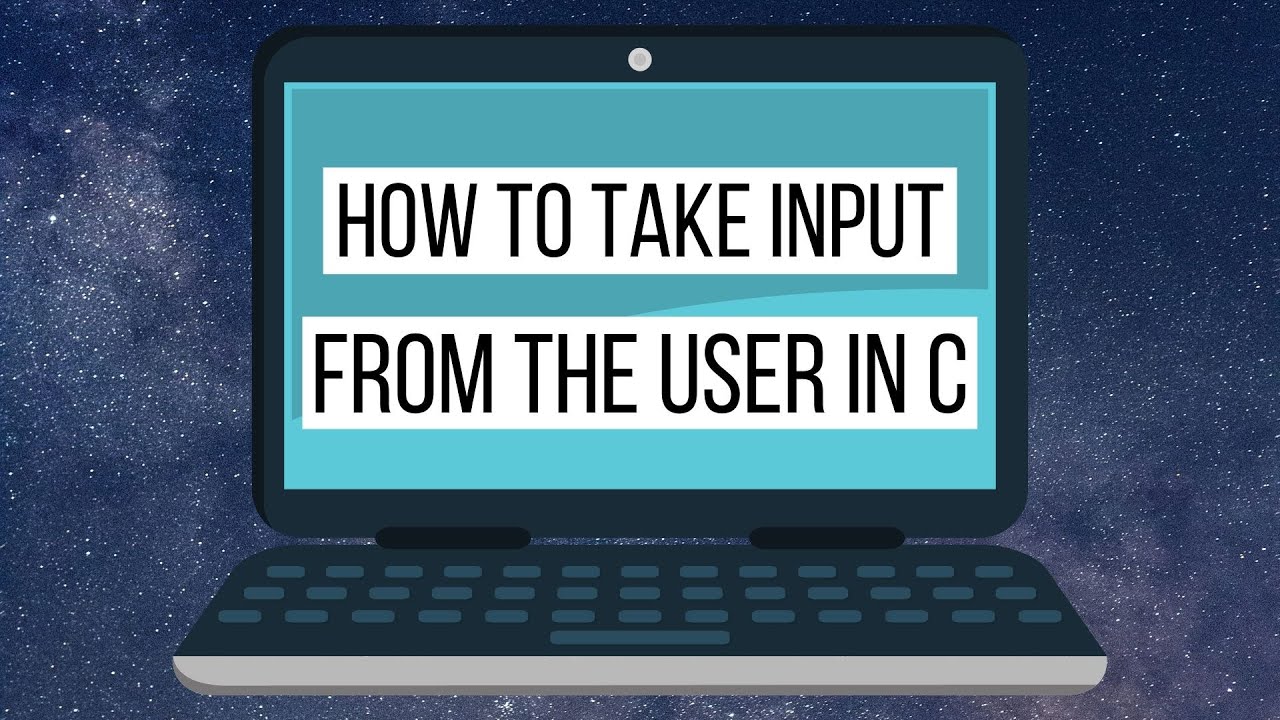
C Program to take Integer Input from the User!!! YouTube
David Egan Objective: get an integer input from stdin. At first glance, scanf () looks like a reasonable way to collect integer input. The function allows formatted input to be collected (it's name comes from the words "scan formatted"). To scan integer input, first declare an integer and pass a pointer to this to scanf:
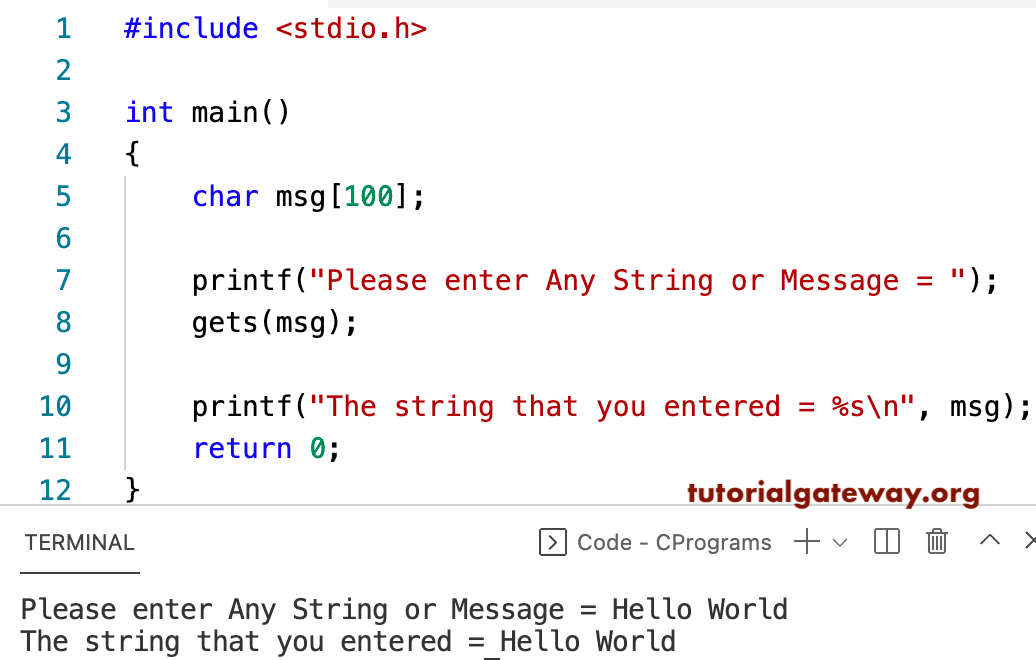
C Program to Read Input and Print String
Use the correct keyword to get user input, stored in the variable x: int x; cout << "Type a number: "; >> ;

C Programming Tutorial User Input & Address Operator Chap2 Part17 Programming tutorial
Here's how you can take input from the user and store it in an array element. // take input and store it in the 3rd element scanf("%d", &mark[2]); // take input and store it in the ith element scanf("%d", &mark[i-1]);. Enter number of elements: 5 Enter number1: 45 Enter number2: 35 Enter number3: 38 Enter number4: 31 Enter number5: 49.
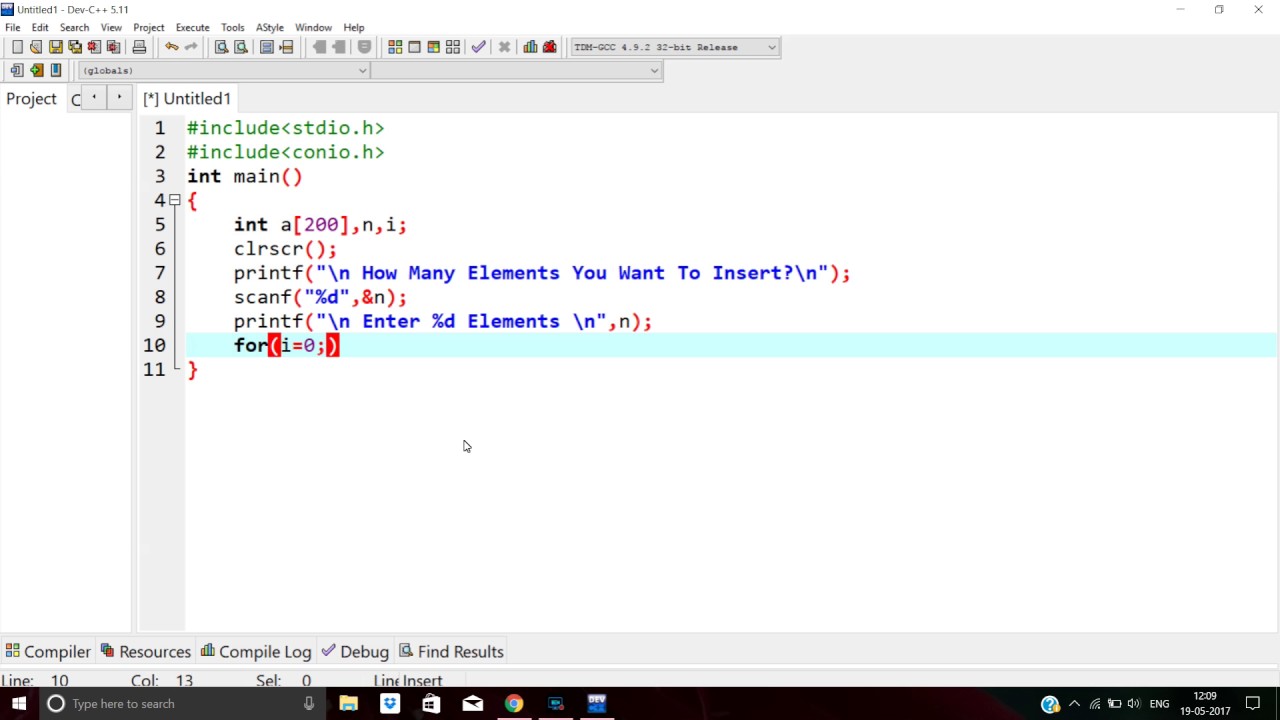
C Program For Array Input Output YouTube
1. Printing Integer values in C Approach: Store the integer value in the variableOfIntType x. Print this value using the printf () method. The printf () method, in C, prints the value passed as the parameter to it, on the console screen. Syntax: printf ("%d", variableOfIntType); Below is the C program to print the integer value: C
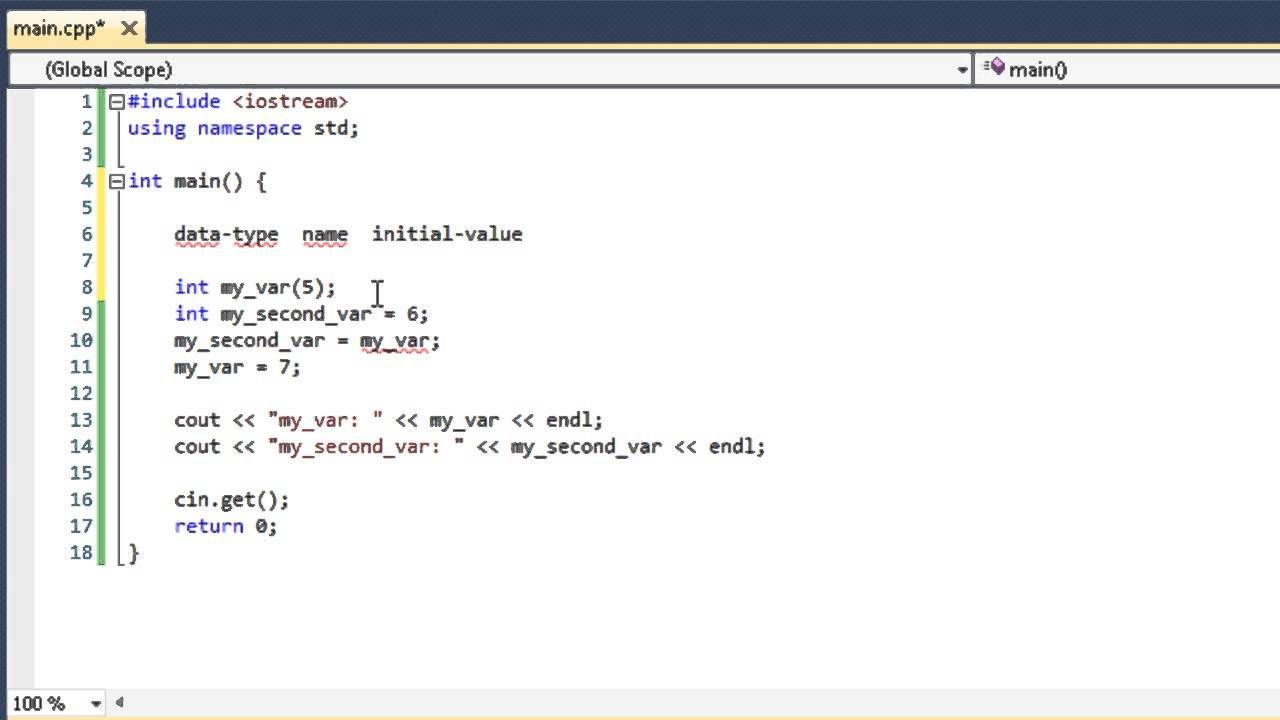
Let's Learn C++ [Lesson 1.3] Integers and Basic Input YouTube
1 Your upper limit, 10,000,000,000 (ten billion), is quite a large number. It won't fit in a 32-bit unsigned integer, you need something larger.
Every Complete C++ Program Must Have a
In this tutorial, we will be making a program on how to take only an Integer value as an input. If the user inputs any value other than an integer, then the program will ask the user to input another integer value till the user inputs an integer value. Program for taking only integer input in C++ #include

Read Integers into C Subsequent calls the method retrieve your input one character at a time
Download the code from GitHub. Here, The num, integerValue and decimalValue are three double variables to hold the user input number, the integer part and the decimal part of the number respectively.; It asks the user to enter a number and reads it in the num variable. We are using the scanf method to read the user input number.; By using the modf function, the program finds the integer and.
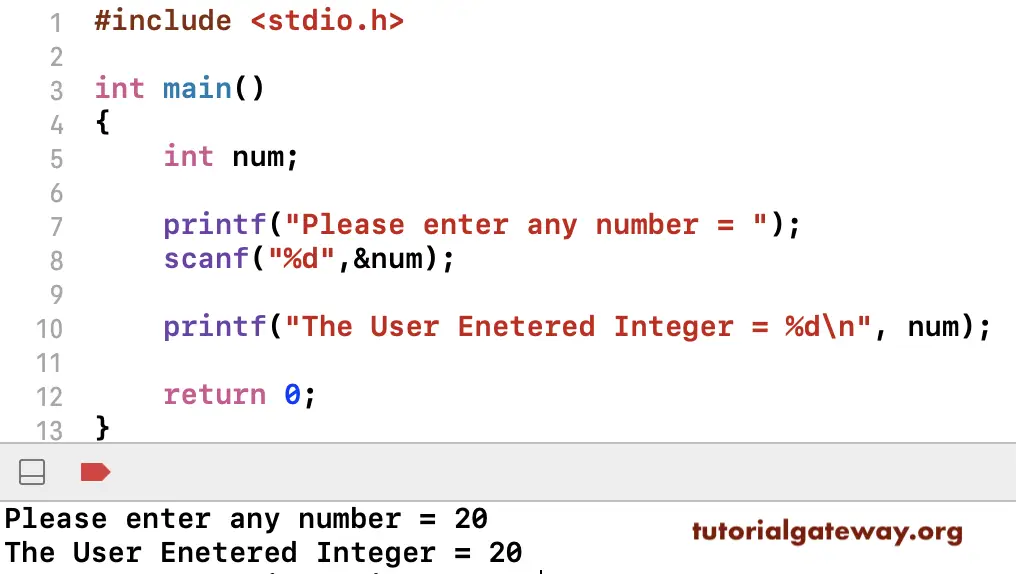
Take Input In C
In this article, we have explained how to take string input in C Programming Language using C code examples. We have explained different cases like taking one word, multiple words, entire line and multiple lines using different functions. Table of contents: String input using scanf Function 1.1. Reading One Word 1.2. Reading Multiple Words 1.3.